Snippet: Google Picker Account
If we want to show a picker with Google accounts, it has become extremely simple with Google Play Services.
Until a few months ago, we would have had to use something like this:
Now we can use new AccountPicker.newChooseAccountIntent() method.
First of all, we need to make sure the Google Play Services client library is being included in the Android's project build path.
Here our code:
There are many advantages:
You can get code from GitHub:
Until a few months ago, we would have had to use something like this:
AccountManager manager = (AccountManager) getSystemService(ACCOUNT_SERVICE); Account[] list = manager.getAccounts(); for(Account account: list) { if(account.type.equalsIgnoreCase("com.google")) { //Do something } }To use this code we need the following permission in your manifest:
Now we can use new AccountPicker.newChooseAccountIntent() method.
First of all, we need to make sure the Google Play Services client library is being included in the Android's project build path.
- Select Project > Properties > Java Build Path > Libraries from the Eclipse menu.
- Click Add External JARs.
- Browse to android-sdk-folder/extras/google/google_play_services/libproject/google-play-services_lib/libs, select google-play-services.jar and click OK.
- In the Order and Export tab, make sure the checkbox by the google-play-services.jar file is checked.
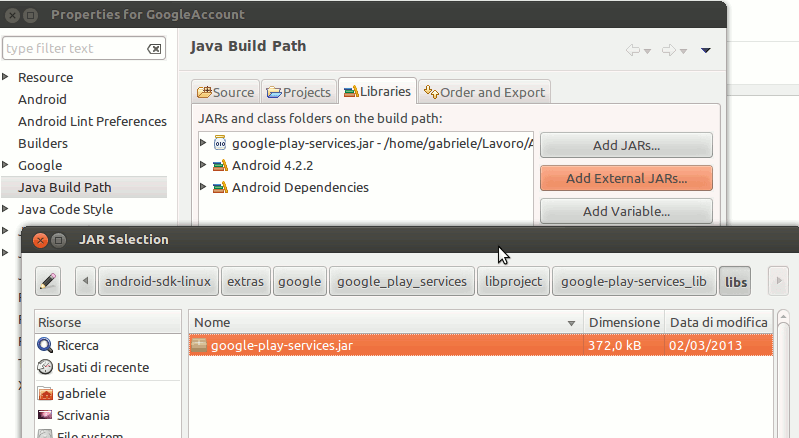
Here our code:
Intent googlePicker =AccountPicker.newChooseAccountIntent(null,null, new String[]{GoogleAuthUtil.GOOGLE_ACCOUNT_TYPE},true,null,null,null,null) ; startActivityForResult(googlePicker,PICK_ACCOUNT_REQUEST); @Override protected void onActivityResult(final int requestCode, final int resultCode, final Intent data) { if (requestCode == PICK_ACCOUNT_REQUEST && resultCode == RESULT_OK) { String accountName = data.getStringExtra(AccountManager.KEY_ACCOUNT_NAME); Toast.makeText(this,"Account Name="+accountName, 3000).show(); } }In our code we use:
GoogleAuthUtil.GOOGLE_ACCOUNT_TYPE
argument as the value for the allowableAccountTypes argument to take only Google Accountstrue
for alwaysPromptForAccount argument to show account chooser screen anyway
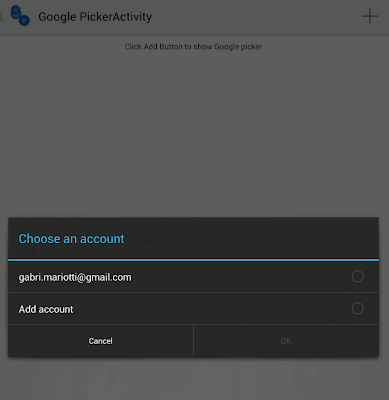
There are many advantages:
- it is very simple to use
- it standardizes user experience with a pick list with same look and feel in all apps
- it doesn't require user-permission
You can get code from GitHub:
This comment has been removed by the author.
ReplyDeleteWhen i touch outside of this dialog, it dismiss.
ReplyDeleteHow can i fix this?