How to work with JSON in Android
In this post I'll describe a way to work with JSON in Android.
.
First of all, what is JSON.
JSON is short for JavaScript Object Notation and it is an independent data exchange format.
Data structures in JSON are based on key / value pairs and we can summarize in these points:
If you observe normally JSON data will have square brackets and curly brackets. The difference between [ and { is, the square bracket represents starting of an JSONArray node whereas curly bracket represents JSONObject.
This is an example:
Android includes the json.org libraries which allow to work easily with JSON files.
Create a JSONObject:
To put a specific value
To create a specific array
To put a specific array in a JSONObject
Create a JSONObject from a JSON String:
To get a specific string from JSON Object
To get a specific int
To get a specific array
To get the items from the array
Now we have all the tools to build an example.
In this example, we'll use a entity.
We can create our MyObject instance:
Now we can write a JSON String from our instance:
* {"id":10,"listMessages":[
* {"value":1,"text":"value","id_message":0},
* {"value":11,"text":"value","id_message":1},
* {"value":21,"text":"value","id_message":2},
* {"value":31,"text":"value","id_message":3},
* {"value":41,"text":"value","id_message":4}
* ]
* ,"name":"myname"}
If you want to parse your JSON String you can use:
Of course you can use external Libraries like GSON.
In this case you have to do these step:
You can get code from GitHub:
.
First of all, what is JSON.
JSON is short for JavaScript Object Notation and it is an independent data exchange format.
Data structures in JSON are based on key / value pairs and we can summarize in these points:
- JSON Object : { string : value , .... }
- JSON Array : [ value , value .....]
- value : string || number || object || array || true || false, || null
If you observe normally JSON data will have square brackets and curly brackets. The difference between [ and { is, the square bracket represents starting of an JSONArray node whereas curly bracket represents JSONObject.
This is an example:
{ "id":10, "name":"myname", "listMessages": [ { "value":1, "text":"value", "id_message":0 }, { "value":11,"text":"value","id_message":1} ] }
Android includes the json.org libraries which allow to work easily with JSON files.
Create a JSONObject:
JSONObject jsonObject = new JSONObject();
To put a specific value
jsonObject.put("id", 10);
jsonObject.put("name", "myname");
To create a specific array
JSONArray jsonArrayMessages = new JSONArray();
JSONObject jsonMessage = new JSONObject();
jsonMessage.put("id_message", 1);
jsonMessage.put("value", 10);
jsonArrayMessages.put(jsonMessage);
To put a specific array in a JSONObject
jsonObject.put("listMessages", jsonArrayMessages);
Create a JSONObject from a JSON String:
JSONObject jsonObject = new JSONObject(result);
To get a specific string from JSON Object
String name = jsonObject.getString("name");
To get a specific int
int id = jsonObject.getInt("id");
To get a specific array
JSONArray jArray = jsonObject.getJSONArray("listMessages");
To get the items from the array
JSONObject msg = jArray.getJSONObject(1);
int id_message = msg.getInt("id_message");
Now we have all the tools to build an example.
In this example, we'll use a entity.
public static class MyObject { private int id; private String name; private List listMessages; } public static class MyObjectMessage { private int id_message; private int value; private String text; }
We can create our MyObject instance:
public static MyObject createMyObject() { MyObject myObj = new MyObject(); myObj.setId(10); myObj.setName("myname"); Listmessages = new ArrayList (); for (int i = 0; i < 5; i++) { MyObjectMessage objMessage = new MyObjectMessage(); objMessage.setId_message(i); objMessage.setValue(i * 10 + 1); objMessage.setText("value"); messages.add(objMessage); } myObj.setListMessages(messages); return myObj; }
Now we can write a JSON String from our instance:
public static JSONObject write() { MyObject myObj = createMyObject(); if (myObj != null) { try { JSONObject jsonObject = new JSONObject(); jsonObject.put("id", myObj.getId()); jsonObject.put("name", myObj.getName()); JSONArray jsonArrayMessages = new JSONArray(); ListHere you can find output:messages = myObj.getListMessages(); if (messages != null) { for (MyObjectMessage objMsg : messages) { JSONObject jsonMessage = new JSONObject(); jsonMessage.put("id_message", objMsg.getId_message()); jsonMessage.put("value", objMsg.getValue()); jsonMessage.put("text", objMsg.getText()); jsonArrayMessages.put(jsonMessage); } } jsonObject.put("listMessages", jsonArrayMessages); return jsonObject; } catch (JSONException je) { Log.e(TAG, "error in Write", je); } } return null; }
* {"id":10,"listMessages":[
* {"value":1,"text":"value","id_message":0},
* {"value":11,"text":"value","id_message":1},
* {"value":21,"text":"value","id_message":2},
* {"value":31,"text":"value","id_message":3},
* {"value":41,"text":"value","id_message":4}
* ]
* ,"name":"myname"}
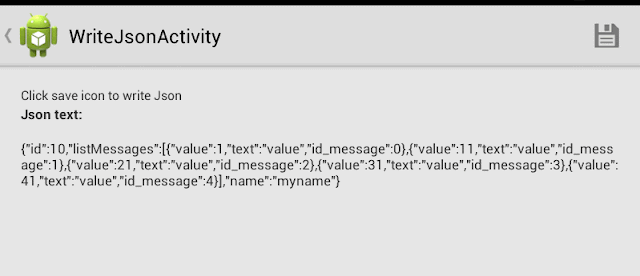
If you want to parse your JSON String you can use:
public static MyObject parse(String jsonString) { MyObject myobj = null; if (jsonString != null) { try { JSONObject jsonObject = new JSONObject(jsonString); myobj = new MyObject(); myobj.setId(jsonObject.getInt("id")); myobj.setName(jsonObject.getString("name")); Listmessages = new ArrayList (); JSONArray msgs = (JSONArray) jsonObject.get("listMessages"); for (int i = 0; i < msgs.length(); i++) { JSONObject json_message = msgs.getJSONObject(i); if (json_message != null) { MyObjectMessage objMsg = new MyObjectMessage(); objMsg.setId_message(json_message.getInt("id_message")); objMsg.setValue(json_message.getInt("value")); objMsg.setText(json_message.getString("text")); messages.add(objMsg); } } myobj.setListMessages(messages); } catch (JSONException je) { Log.e(TAG, "error while parsing", je); } } return myobj; }
Of course you can use external Libraries like GSON.
In this case you have to do these step:
- Download GSON library
- Add library to your project
- Modify our code
public static String writeGson() { MyObject myObj = createMyObject(); if (myObj != null) { Gson gson = new Gson(); String objJson = gson.toJson(myObj); return objJson; } return null; }If you want to parse your JSON String you can use:
public static MyObject parseGson(String jsonString) { MyObject myobj = null; if (jsonString != null) { Gson gson = new Gson(); myobj = gson.fromJson(jsonString, MyObject.class); } return myobj; }
You can get code from GitHub:
Hi admin Fantastic blog! Do you have any tips and hints for aspiring writers? I’m planning to start my own website soon but I’m a little lost on everything. دمج pdf
ReplyDelete