Quick tips for ListView: build a layout like a chat with TranscriptMode and StackFromBottom
Our purpose is to create a very simple layout with a ListView that looks like a chat.
Something like this:
It is very easy.
Let's take a common ListView in a RelativeLayout.
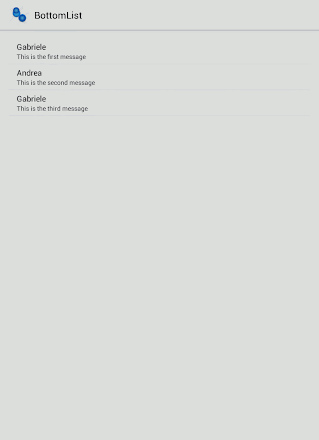
This is still not what we wuold like to achieve.
Now we'll add an xml attribute to ListView:android:stackFromBottom="true".
In this way the ListView stacks its content from the bottom.
We'll optimize the scroll with another xml attribute:
android:transcriptMode="alwaysScroll"
In this way the list will automatically scroll to the bottom.
Ok, with two simple attributes we obtained the list.
Now we'll add a EditText at the bottom, to complete our layout.
Sure it's a small example, the next step is to add a small animation.
You can get code from GitHub (built with AndroidStudio):
Something like this:
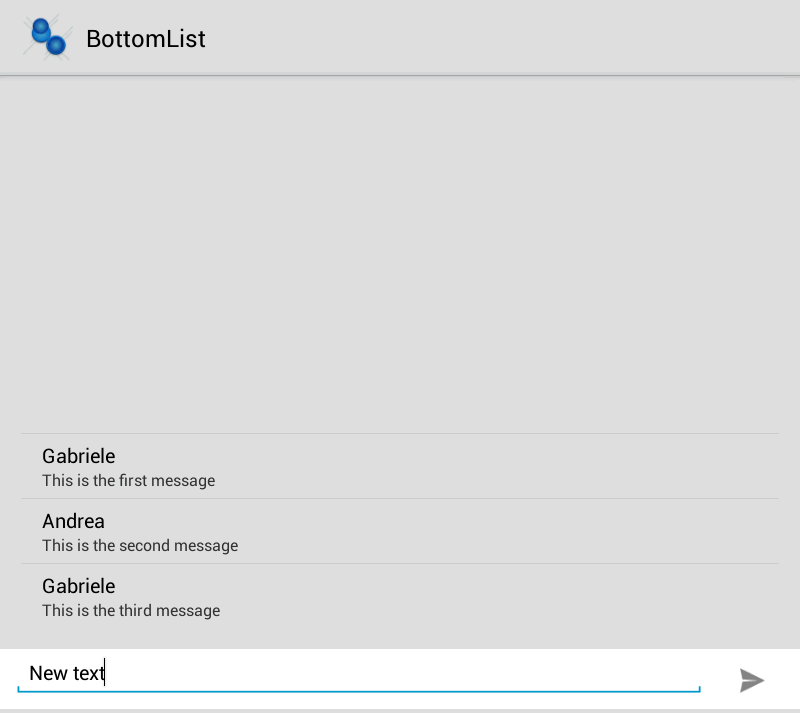
Let's take a common ListView in a RelativeLayout.
Let's populate a simple Adapter, and run it.
public class MainActivity extends ListActivity { private ViewHolderAdapter mAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mAdapter = ListHelper.buildViewHolderAdapter(this, R.layout.list_item); setListAdapter(mAdapter); } }
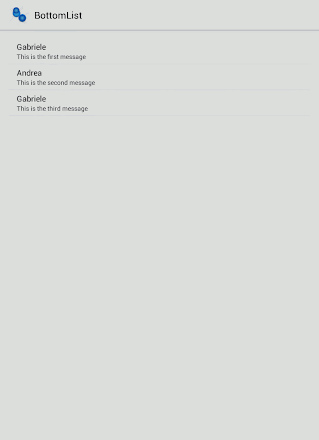
This is still not what we wuold like to achieve.
Now we'll add an xml attribute to ListView:android:stackFromBottom="true".
In this way the ListView stacks its content from the bottom.
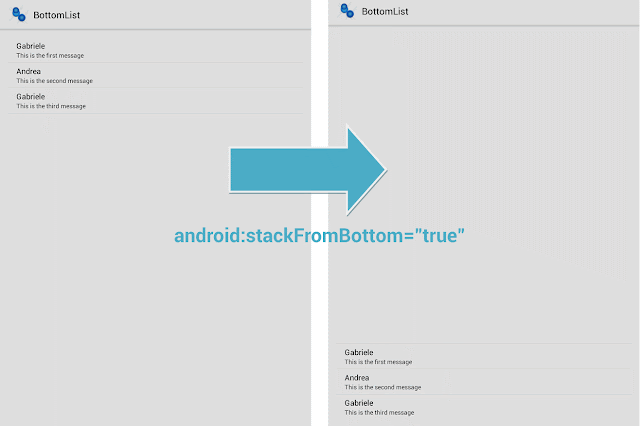
We'll optimize the scroll with another xml attribute:
android:transcriptMode="alwaysScroll"
In this way the list will automatically scroll to the bottom.
Ok, with two simple attributes we obtained the list.
Now we'll add a EditText at the bottom, to complete our layout.
In our Activity, we'll add a Listener to ImageButton, to add items.
That's it!public class MainActivity extends ListActivity { private EditText mNewMessage; private ImageButton mNewMessageSend; private ViewHolderAdapter mAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mAdapter = ListHelper.buildViewHolderAdapter(this, R.layout.list_item); setListAdapter(mAdapter); mNewMessage = (EditText) findViewById(R.id.newmsg); mNewMessageSend = (ImageButton) findViewById(R.id.newmsgsend); if (mNewMessageSend!=null){ mNewMessageSend.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { addItem(); } }); } } private void addItem() { MyObj obj = new MyObj("Gabriele",mNewMessage.getText().toString()); mAdapter.add(obj); mAdapter.notifyDataSetChanged(); } }
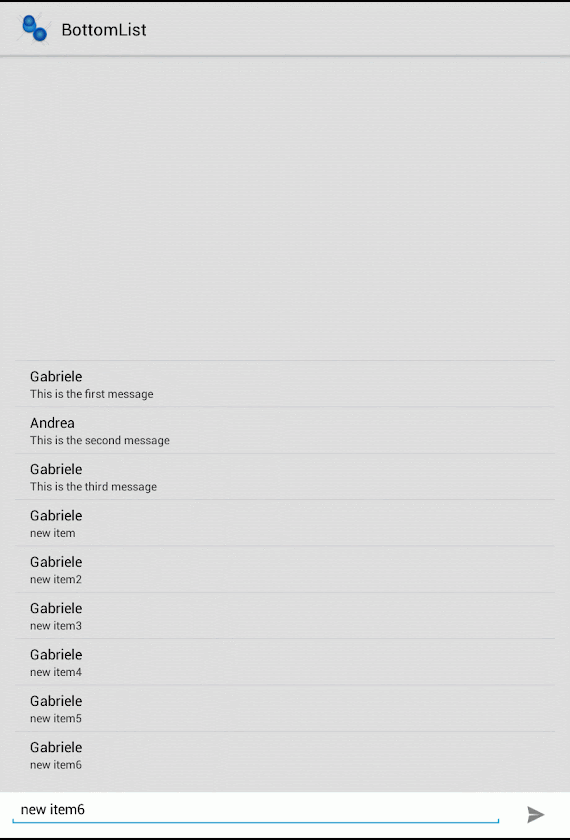
Sure it's a small example, the next step is to add a small animation.
You can get code from GitHub (built with AndroidStudio):
Lovely bllog you have
ReplyDelete